oauth
/oauth
OAuth guide
UltraCart allows you to build applications that communicate with the UltraCart service on behalf of users. You will need to have each use authenticate, verify their identity, with UltraCart and then grant permission to your application to access data on their UltraCart account.
UltraCart uses OAuth 2, an open specification, for third party application authentication. Once a user completes the OAuth process, an access token is returned to your application. The access token is a long string generated by UltraCart that you'll need to send with each subsequent API request to uniquely identify both your application and the end user.
There are several important benefits that lead us to select OAuth:
- Most importantly, your application does not need to store or transmit the UltraCart user's password.
- OAuth allows the user to authorize a limited set of permissions to your application.
- Users can revoke access to an individual application at any time.
- The protocol is an industry (IETF) standard that has had multiple revisions and security reviews.
This makes OAuth a safer and more secure form of API authorization for your users.
Setting up your application
Before you can get started, you'll need to register your application with UltraCart under:
Configuration -> Back Office -> Authorized Applications -> Developer Applications
Under this management screen you will be able to configure the application name, developer information, redirect URL, and permissions.
After creating your application, you are ready to set-up the authorization process in your application. The UltraCart SDKs contain the API methods below to assist in the OAuth flow.
OAuth 2 flow
Here's a simple diagram of the OAuth 2 flow - the process for authorizing your users with UltraCart.
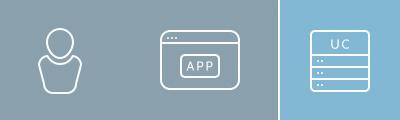
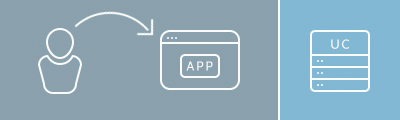
User App: Load my item information from UltraCart.
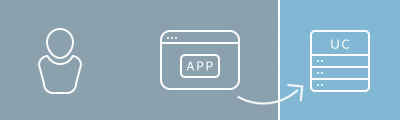
App UltraCart: User would like to load item information from UltraCart
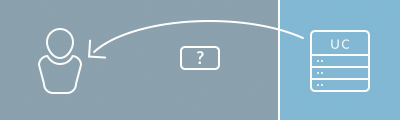
UltraCart User: User would like allow the application to load your item information?
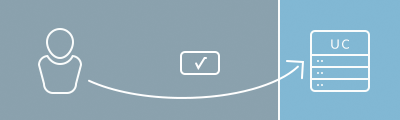
User UltraCart: Yes, allow the application access to my item information
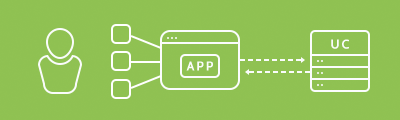
The Application and UltraCart can now send and recieve the users item information.
The high level idea is that the user will be redirected to UltraCart to authorize your application to access their UltraCart data. After the user has approved your application, they'll be sent back to your application with an authorization code. At this point your application will exchange the authorization code for an access token which can be used to make subsequent requests to the UltraCart API (not shown in the diagram above).
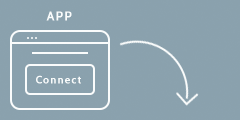
- User presses a “Connect” button
- Your App redirects the user to a UltraCart webpage. /oauth2/authorize?response_type=code
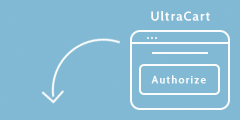
- The user logs into UltraCart and authorizes your app
- UltraCart redirects the user back to your app using the redirect_uri you provided.
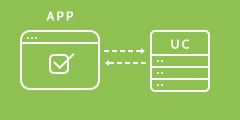
- Your app exchanges the authorization code for a reusable access token (not visible to the user).
If you're building a web application, the first step in the OAuth process is to redirect the user to an UltraCart web page. Typically the user takes some action on your site, such as clicking a "Connect to UltraCart" button, and your application will need to redirect the user to a particular UltraCart authorization URL.
The UltraCart authorization URL is specific to your application and is composed of your client ID, redirect URI, response type, and state (it looks something like
https://secure.ultracart.com/admin/v2/oauth/authorize?client_id=...&redirect_uri=...&state=...&response_type=code). The complete /authorize
URL is documented below. You will need to generate this complete
URL and have the user click on it to start the process. Make sure you are careful about properly URL encoding each parameter value when building the URL.
At this point in the flow, the user will need to login to UltraCart. Once the user is logged into UltraCart, they will be presented with a screen to authorize your application to access their UltraCart data.
TODO: UltraCart authorize screen
After the user approves your application, they'll be redirected from UltraCart back to your application using the redirect URI provided in the application configuration or UltraCart authorization URL. For security, this redirect URI must match one of the redirect URIs you have specified for your application and also contain a secret state parameter which will be returned in the redirect.
The redirect back to your application will include an authorization code from UltraCart. Your application will then need to exchange the authorization code for a re-usable access token immediately.
The exchange takes place in the background of your application using a call to the /token
API documented below.
The access token is the important credential that is needed to make successful UltraCart API calls. You'll want to store this within your application securely and it should not be displayed to the end user.
Now your application is authorized to use the UltraCart API on behalf of your user. When you'd like to make API calls to UltraCart, simply include the authorization header,
Authorization: Bearer <YOUR_ACCESS_TOKEN_HERE>
, with each request.